NOTE: Better to watch the video on youtube and viewed full screen.
In this project I take a Nerf Maverick and use it as my mouse for my laptop. This was easier than I thought it would be. It took about two days to do, and I use python for the computer interface. This is much like the Nerf gun for the Wii. You could do the same thing with the gun for the Wii and connect your Wiimote to the PC via bluetooth. That way uses PyGlove as the emulator.
The first step was to find a way to “click” with the trigger of the gun. After opening the Maverick I found the trigger when pulled touches another part inside the gun. This made it easy to wrap some wires around the two parts so it would act as a button. (Figure 1-1; Figure 1-2)
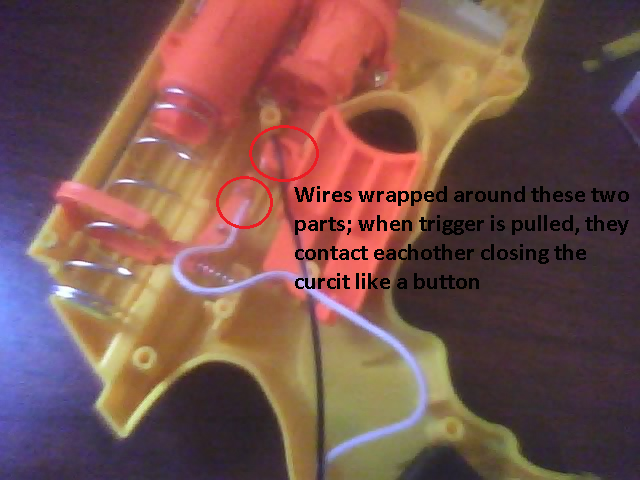
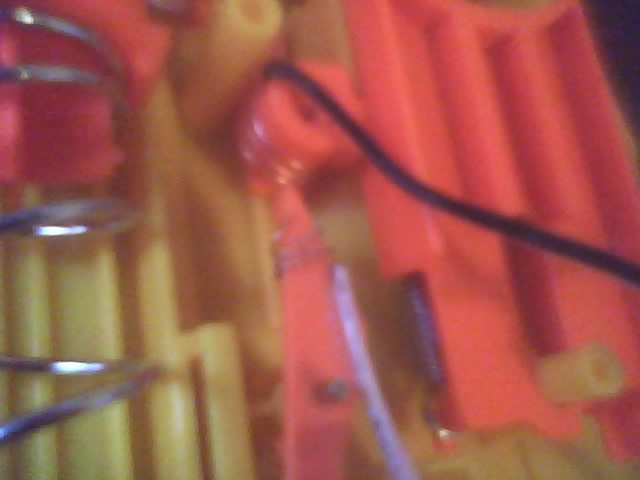
Next was to find a spot for the 9v so I could power the ArduinoBT and the circuits. I used a soldering knife to cut out a spot. It is in the handle of the gun kind of like a clip. (Figure 1-3; Figure 1-4)
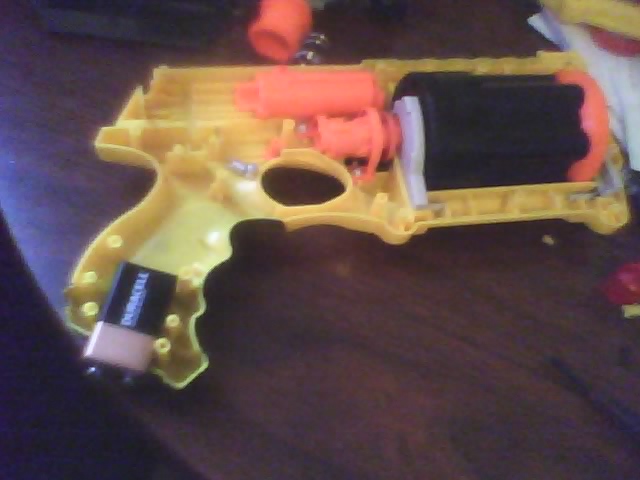
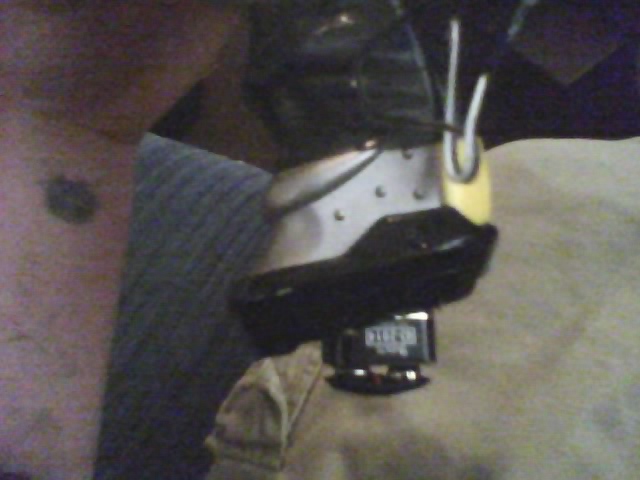
After I got the circuits working it was time for the code. I used Python2.6 and an infrared tracking library I wrote to map the mouse movements, and when the trigger is pulled, it sends a message to the COM port to tell python to click the left mouse button.
//nerf.pde int trigPin = 13; int pulled = 0; void setup() { Serial.begin(115200); pinMode(trigPin, INPUT); } void loop() { pulled = digitalRead(trigPin); if (pulled == HIGH) { Serial.println("d"); } else { Serial.println("u"); } }
This is the script to read the arduino and map the mouse. IRClass is found in my blog. It is my first post. The variable “c” is used to make sure there aren’t any accidental double clicks and it allows the “drag-n-drop” feature.
import serial import win32api import win32con from ctypes import * import IRClass ir = IRClass.IR() s = serial.Serial('COM9',baudrate=115200) user = windll.user32 #These functions control the mouse clicks. def lUP(): win32api.mouse_event(win32con.MOUSEEVENTF_LEFTUP,0,0) def lDOWN(): win32api.mouse_event(win32con.MOUSEEVENTF_LEFTDOWN,0,0) c = 0 while 1: x,y = ir.xySinglePos(scale=(1440,900)) ir.user.SetCursorPos(x,y) s.flushInput() buff = s.readline() if "d" in buff and c == 0: lDOWN() c += 1 elif "u" in buff: lUP() c = 0
These are pictures of the completed gun.
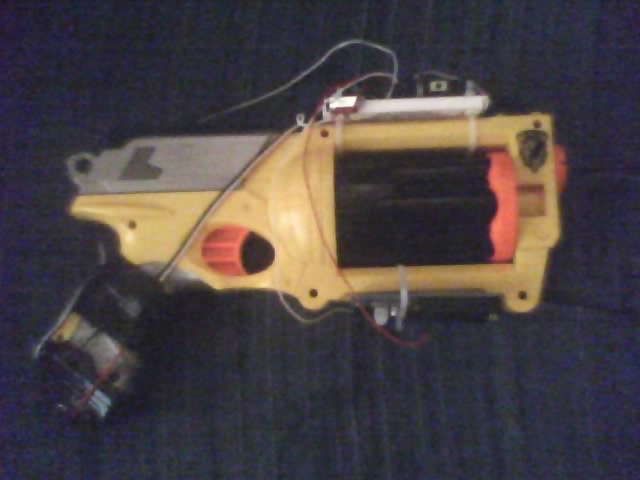
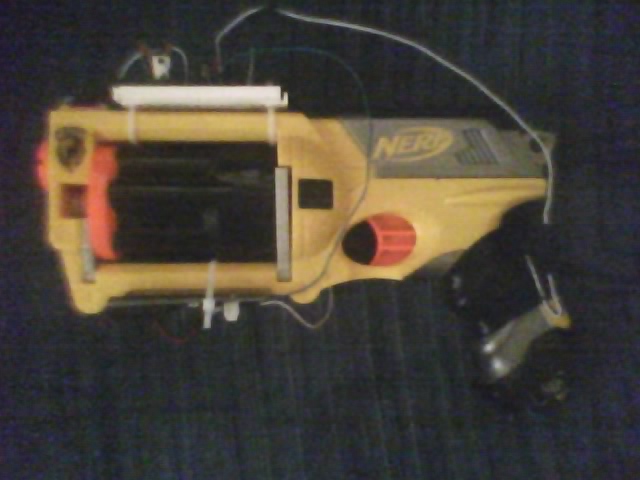
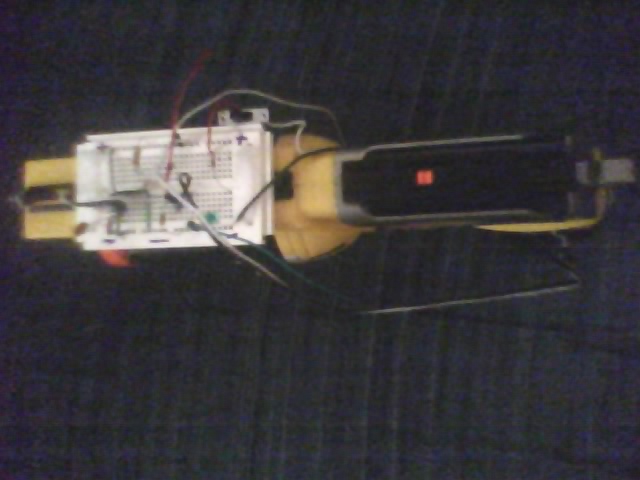
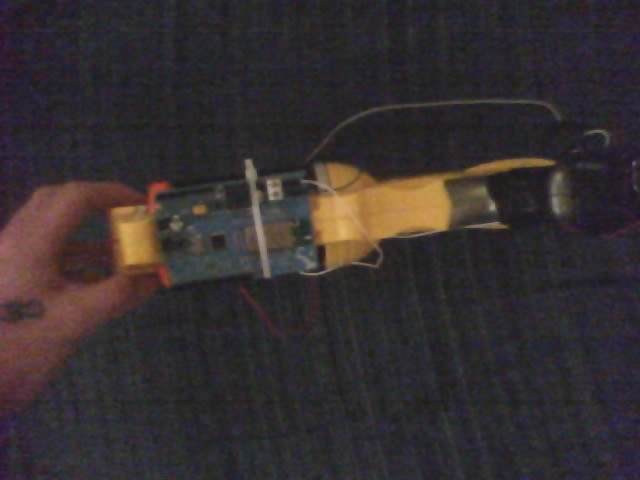
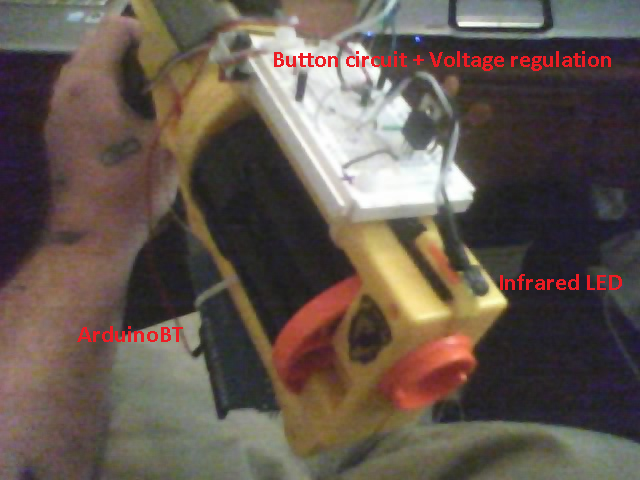