I thought it would be a good time to share what I am currently working on. A friend from work and I are building costumes. Not necessarily for Halloween, but because I’ve always wanted a predator outfit. Sure you can buy them online, but not with the features I’m adding. I am going to mount a webcam on the mask and put some video glasses in it as well. Something like these:
I might have two webcams, so one can be infrared and the other normal. Making a webcam into an infrared cam is really easy. There are plenty of resources on doing it:
- http://www.hoagieshouse.com/IR/
- http://www.instructables.com/id/Infrared-IR-Webcam/
- http://hackaday.com/2005/03/14/make-an-infrared-webcam/
The code is really simple (so far). I have been thinking about changing the pixel colors to mimic thermal vision. Anything moving is highlighted between yellow and red, pending on how high the deferance is. That is still an idea, and I am currently at a block. I will post updates when they come.
Requirements:
- Pygame for video feeed : http://pygame.org/news.html
- VideoCapture for handle to webcam : http://videocapture.sourceforge.net/
- PIL for image processing : http://www.pythonware.com/products/pil/
#load what we need from VideoCapture import Device import pygame, Image, sys, ImageChops, time from pygame.locals import * #grab handle cam = Device() #find out the resolution res = cam.getImage() res = res.size #initiate pygame pygame.init() screen = pygame.display.set_mode(res) pygame.display.set_caption("Predator Vision: by Tech B") while 1: #check for quit for event in pygame.event.get(): if event.type == pygame.QUIT: sys.exit() #grab two images im1 = cam.getImage() #you will need to wait a sec before taking another image #if not, the screen will remain black and buggy time.sleep(.2) im2 = cam.getImage() #im2-im1 per pixel diff = ImageChops.difference(im1,im2) #convert image to pygame type and then display shot = pygame.image.frombuffer(diff.tostring(), res, "RGB") screen.blit(shot,(0,0)) pygame.display.flip()
::UPDATE::
I can now change the color of the “movement” pixels.
Add this code between
diff = ImageChops.difference(im1,im2)
and
shot = pygame.image.frombuffer(diff.tostring(), res, “RGB”)
n = diff.load() for x in range(0,res[0]): for y in range(0,res[1]): if n[x,y] > (25,25,25): n[x,y] = (255,0,0) else: n[x,y] = (0,0,0)
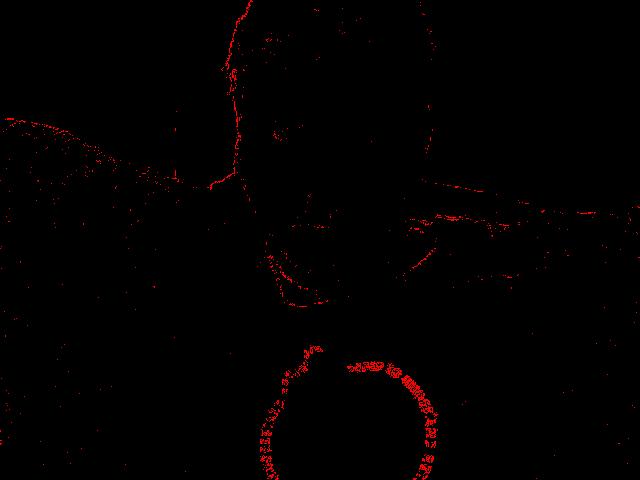
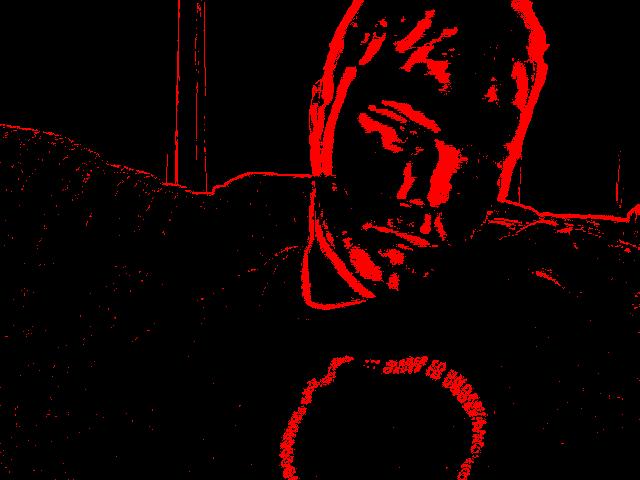